프로그램 개요
Android 9(API Level 28, Pie)에서 새로 추가된 기능으로 핸드폰을 Bluetooth HID로 사용할 수 있도록 지원하고 있습니다. 이 기능을 통하여 핸드폰을 키보드, 마우스, 게임 패드 등의 입력장비로 사용 가능 합니다.
프로그램 작동 원리
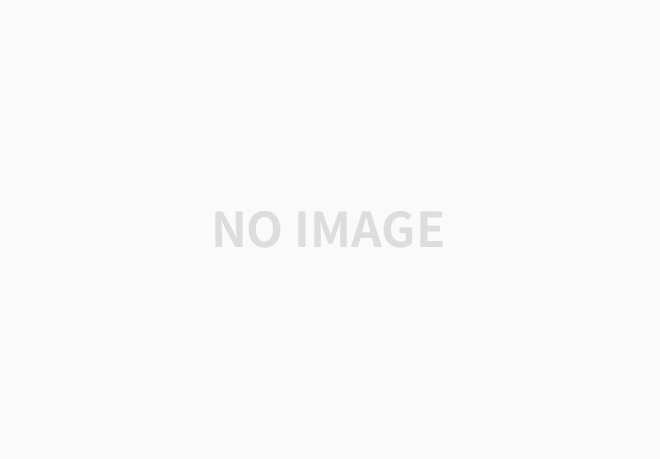
Bluetooth 설정하기
블루투스 기기의 정보를 취득 후에는 활성화한 HID 서비스와 통신할 수 있도록 프록시(BluetoothHidDevice)를 가져와야 합니다.
처음으로 블루투스를 활성화 하고 블루트스 어댑터를 가져옵니다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
private BluetoothAdapter mBtAdapter = BluetoothAdapter.getDefaultAdapter();
@Override
protected void onResume() {
super.onResume();
// Get bluetooth enabled before continuing
if (!mBtAdapter.isEnabled()) {
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
} else {
btListDevices();
}
}
|
cs |
그 다음으로 페어링된 목록을 가져옵니다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
|
private void btListDevices() {
getProxy(); // need bluetooth to have been enabled first
Set<BluetoothDevice> pairedDevices = mBtAdapter.getBondedDevices();
// Add devices to adapter
List<String> names = new ArrayList<>();
// add empty
names.add("(disconnected)");
mDevices.add(null);
for (BluetoothDevice btDev : pairedDevices) {
names.add(btDev.getName());
mDevices.add(btDev);
}
Spinner btList = findViewById(R.id.devices);
ArrayAdapter<String> adapter = new ArrayAdapter<>(
this, android.R.layout.simple_spinner_item, names);
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
btList.setAdapter(adapter);
btList.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position, long id) {
BluetoothDevice dev = mDevices.get(position);
Log.d(TAG, "onItemSelected(): " + dev + " " + position + " " + id);
btConnect(dev);
}
@Override
public void onNothingSelected(AdapterView<?> parent) {
//TODO handle this
}
});
}
|
cs |
getProfileProxy
HID 서비스와 통신할 수 있도록 Profile과 연결된 프로필 프록시를 요청하고 저장합니다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
mBtAdapter.getProfileProxy(this, new BluetoothProfile.ServiceListener() {
@Override
@SuppressLint("NewApi")
public void onServiceConnected(int profile, BluetoothProfile proxy) {
if (profile == BluetoothProfile.HID_DEVICE) {
Log.d(TAG, "Got HID device");
mBtHidDevice = (BluetoothHidDevice) proxy;
...
mBtHidDevice.registerApp();
}
}
@Override
public void onServiceDisconnected(int profile) {
if (profile == BluetoothProfile.HID_DEVICE) {
Log.d(TAG, "Lost HID device");
}
}
}, BluetoothProfile.HID_DEVICE);
|
cs |
registerApp
HID 장치로 사용할 애플리케이션을 등록합니다.
HID 장치에 대한 연결은 애플리케이션이 등록 된 경우에만 가능합니다. 또한, 한 번에 하나의 응용 프로그램 만 등록 할 수 있습니다. HidDevice을 사용하는 앱을 복수로 사용 할 수 없습니다.
1
2
3
4
5
|
public boolean registerApp (BluetoothHidDeviceAppSdpSettings sdp,
BluetoothHidDeviceAppQosSettings inQos,
BluetoothHidDeviceAppQosSettings outQos,
Executor executor,
BluetoothHidDevice.Callback callback)
|
cs |
sdp
Bluetooth HID 장치 응용 프로그램에 대한 SDP (서비스 검색 프로토콜) 설정을 나타냅니다.
BluetoothHidDevice 프레임 워크는 앱 등록 중에 SDP 레코드를 추가하므로 Android 장치가 Bluetooth HID 장치로 검색 될 수 있습니다.
1
2
3
4
5
|
public BluetoothHidDeviceAppSdpSettings (String name,
String description,
String provider,
byte subclass,
byte[] descriptors)
|
cs |
이름, 설명, 공급자, 하위클래스, Bluetooth HID 장치 설명
Descriptors
사용할 HID 기기의 descriptor를 설정 합니다.
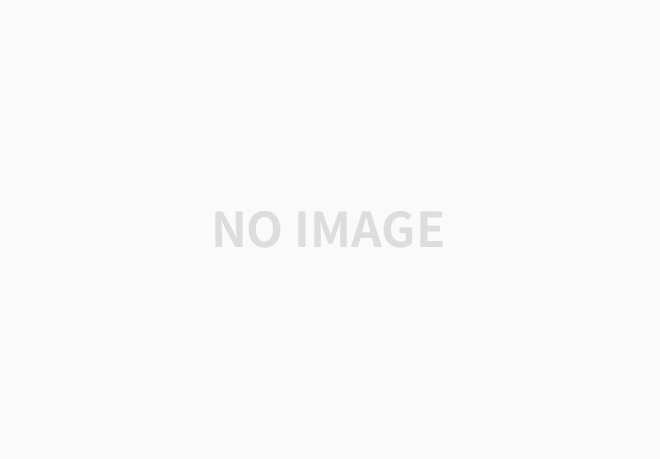
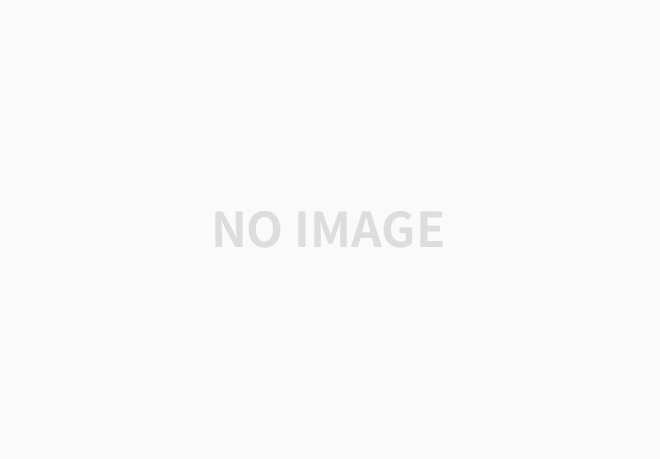
다음 글에서는 블루투스를 연결하고 연결된 장비로 어떻게 input을 보내는지 설명하겠습니다.
'프로젝트 > Android Bluetooth HID' 카테고리의 다른 글
[Android] Bluetooth HID 키보드 앱 만들기 2. Bluetooth 연결/전송 (0) | 2021.03.04 |
---|
댓글